iStringArray Struct Reference
[Utilities]
This is an SCF-compatible interface for csStringArray. More...
#include <iutil/stringarray.h>
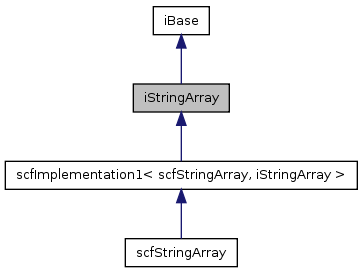
Public Member Functions | |
virtual size_t | Contains (const char *str, bool case_sensitive=true) const =0 |
Alias for Find() and FindCaseInsensitive(). | |
virtual bool | DeleteIndex (size_t n)=0 |
Delete string n from the array. | |
virtual void | Empty ()=0 |
Remove all strings from the array, but does not release the memory allocated. | |
virtual size_t | Find (const char *value) const =0 |
Find a string, case-sensitive. | |
virtual size_t | FindCaseInsensitive (const char *value) const =0 |
Find a string, case-insensitive. | |
virtual size_t | FindSortedKey (const char *value) const =0 |
Find an element based on some key, using a comparison function. | |
virtual char const * | Get (size_t n) const =0 |
Get a particular string from the array. | |
virtual size_t | GetSize () const =0 |
Get array length. | |
virtual bool | Insert (size_t n, char const *value)=0 |
Insert a string before entry n in the array. | |
virtual bool | IsEmpty () const =0 |
Return true if the array is empty. | |
virtual char * | Pop ()=0 |
Pop an element from tail end of array. | |
virtual void | Push (const char *value)=0 |
Push a string onto the stack. | |
virtual void | Put (size_t n, const char *value)=0 |
Insert or reset the element with index n . | |
virtual void | Sort (bool case_sensitive=true)=0 |
Sort array. |
Detailed Description
This is an SCF-compatible interface for csStringArray.
Definition at line 33 of file stringarray.h.
Member Function Documentation
virtual size_t iStringArray::Contains | ( | const char * | str, | |
bool | case_sensitive = true | |||
) | const [pure virtual] |
Alias for Find() and FindCaseInsensitive().
- Parameters:
-
str String to look for in array. case_sensitive If true, consider case when performing comparison. (default: yes)
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- Works with sorted and unsorted arrays, but FindSortedKey() is faster on sorted arrays.
- Some people find Contains() more idiomatic than Find().
Implemented in scfStringArray.
virtual bool iStringArray::DeleteIndex | ( | size_t | n | ) | [pure virtual] |
Delete string n
from the array.
Implemented in scfStringArray.
virtual void iStringArray::Empty | ( | ) | [pure virtual] |
Remove all strings from the array, but does not release the memory allocated.
Implemented in scfStringArray.
virtual size_t iStringArray::Find | ( | const char * | value | ) | const [pure virtual] |
Find a string, case-sensitive.
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- Works with sorted and unsorted arrays, but FindSortedKey() is faster on sorted arrays.
Implemented in scfStringArray.
virtual size_t iStringArray::FindCaseInsensitive | ( | const char * | value | ) | const [pure virtual] |
Find a string, case-insensitive.
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- Works with sorted and unsorted arrays, but FindSortedKey() is faster on sorted arrays.
Implemented in scfStringArray.
virtual size_t iStringArray::FindSortedKey | ( | const char * | value | ) | const [pure virtual] |
Find an element based on some key, using a comparison function.
- Returns:
- csArrayItemNotFound if not found, else item index.
- Remarks:
- The array must be sorted.
Implemented in scfStringArray.
virtual char const* iStringArray::Get | ( | size_t | n | ) | const [pure virtual] |
Get a particular string from the array.
Implemented in scfStringArray.
virtual size_t iStringArray::GetSize | ( | ) | const [pure virtual] |
Get array length.
Implemented in scfStringArray.
virtual bool iStringArray::Insert | ( | size_t | n, | |
char const * | value | |||
) | [pure virtual] |
Insert a string before entry n
in the array.
Implemented in scfStringArray.
virtual bool iStringArray::IsEmpty | ( | ) | const [pure virtual] |
Return true if the array is empty.
- Remarks:
- Rigidly equivalent to
return GetSize() == 0
, but more idiomatic.
Implemented in scfStringArray.
virtual char* iStringArray::Pop | ( | ) | [pure virtual] |
Pop an element from tail end of array.
- Remarks:
- Caller is responsible for invoking delete[] on the returned string when no longer needed.
Implemented in scfStringArray.
virtual void iStringArray::Push | ( | const char * | value | ) | [pure virtual] |
Push a string onto the stack.
Implemented in scfStringArray.
virtual void iStringArray::Put | ( | size_t | n, | |
const char * | value | |||
) | [pure virtual] |
Insert or reset the element with index n
.
If the size of the array is smaller than n
then it will be resized.
Implemented in scfStringArray.
virtual void iStringArray::Sort | ( | bool | case_sensitive = true |
) | [pure virtual] |
Sort array.
- Parameters:
-
case_sensitive If true, consider case when performing comparison. (default: yes)
Implemented in scfStringArray.
The documentation for this struct was generated from the following file:
- iutil/stringarray.h
Generated for Crystal Space 2.0 by doxygen 1.6.1