csInputBinder Class Reference
Use this class to bind input events (keypress, button press, mouse move, etc. More...
#include <csutil/binder.h>
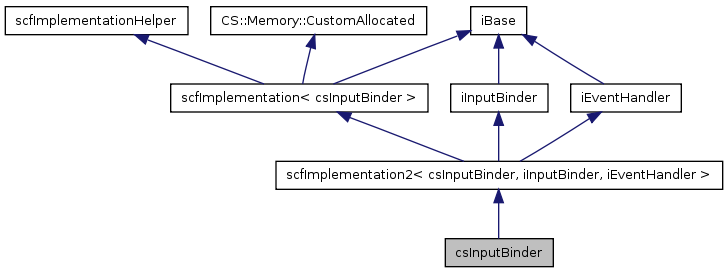
Public Member Functions | |
virtual int | Axis (unsigned cmd) |
Returns the position of the given axis command. | |
virtual void | BindAxis (csInputDefinition const &def, unsigned int cmd, int sensitivity=1) |
Bind an axis motion event to an axis command. | |
virtual void | BindButton (csInputDefinition const &def, unsigned int cmd, bool toggle=false) |
Bind a button event to a button command. | |
virtual bool | Button (unsigned cmd) |
Returns the status of the given button command. | |
csInputBinder (iObjectRegistry *, iBase *parent=0, int btnSize=127, int axisSize=13) | |
Create a new binder with an initial bindings hash size. | |
virtual void | LoadConfig (iConfigFile *, const char *subsection) |
Load bindings from a configuration file. | |
virtual iEventHandler * | QueryHandler () |
Get a pointer to the embedded iEventHander. | |
virtual void | SaveConfig (iConfigFile *, const char *subsection) |
Save bindings to a configuration file. | |
virtual void | UnbindAll () |
Remove all bindings. | |
virtual bool | UnbindAxis (unsigned cmd) |
Remove a binding. | |
virtual bool | UnbindButton (unsigned cmd) |
Remove a binding. | |
Protected Member Functions | |
bool | HandleEvent (iEvent &) |
This is the basic event handling function. |
Detailed Description
Use this class to bind input events (keypress, button press, mouse move, etc.
) to commands which are represented by an unsigned integer. It is up to the application to specify the meaning of a command value.
Example:
enum MyCommand = { Walk, Shoot, Jump, LookX, LookY }; ... csRef<iInputBinder> binder = ...; binder->BindButton (csInputDefinition ("ctrl"), Shoot); binder->BindAxis (csInputDefinition ("mousex"), LookX); ... if (binder->Button (Shoot)) ... else { DoSomething (binder->Axis (LookX), binder->Axis (LookY)); }
Definition at line 57 of file binder.h.
Constructor & Destructor Documentation
csInputBinder::csInputBinder | ( | iObjectRegistry * | , | |
iBase * | parent = 0 , |
|||
int | btnSize = 127 , |
|||
int | axisSize = 13 | |||
) |
Create a new binder with an initial bindings hash size.
For optimum hash storage, size should be a prime number.
Member Function Documentation
virtual int csInputBinder::Axis | ( | unsigned | cmd | ) | [virtual] |
Returns the position of the given axis command.
Implements iInputBinder.
virtual void csInputBinder::BindAxis | ( | csInputDefinition const & | def, | |
unsigned int | cmd, | |||
int | sensitivity = 1 | |||
) | [virtual] |
Bind an axis motion event to an axis command.
- Parameters:
-
def Describes the physical axis to bind to. cmd The ID of the command to bind. sensitivity A multiplier for the axis command.
- Remarks:
- Note that cmd is used as an array index so the numbers you use should be consecutive, starting with 0.
Implements iInputBinder.
virtual void csInputBinder::BindButton | ( | csInputDefinition const & | def, | |
unsigned int | cmd, | |||
bool | toggle = false | |||
) | [virtual] |
Bind a button event to a button command.
- Parameters:
-
def Describes the physical button to bind to. cmd The ID of the command to bind. toggle If true, button status is only toggled on keydown events.
- Remarks:
- Note that cmd is used as an array index so the numbers you use should be consecutive, starting with 0. Also note that there must be a 1:1 mapping between buttons and commands, that is, a button cannot issue multiple commands, and the same command cannot be bound to multiple buttons. If you require this, you must handle it in your application. Binding a button (or command) will remove any previous bindings involving the button or command.
Implements iInputBinder.
virtual bool csInputBinder::Button | ( | unsigned | cmd | ) | [virtual] |
Returns the status of the given button command.
Implements iInputBinder.
bool csInputBinder::HandleEvent | ( | iEvent & | ) | [protected, virtual] |
This is the basic event handling function.
To receive events, a component must implement iEventHandler and register with an event queue using iEventQueue::RegisterListener() and iEventQueue::Subscribe(). The event handler should return true if the event was handled. Returning true prevents the event from being passed along to other event handlers (unless the event's Broadcast flag has been set, in which case it is sent to all subscribers regardless of the return value). If the event was not handled, then false should be returned, in which case other event handlers are given a shot at the event. Do not return true unless you really handled the event and want event dispatch to stop at your handler.
Implements iEventHandler.
virtual void csInputBinder::LoadConfig | ( | iConfigFile * | , | |
const char * | subsection | |||
) | [virtual] |
Load bindings from a configuration file.
Implements iInputBinder.
virtual iEventHandler* csInputBinder::QueryHandler | ( | ) | [inline, virtual] |
Get a pointer to the embedded iEventHander.
- Remarks:
- This class has to be registered with the event queue: EventQueue->RegisterListener(InputBinder->QueryHandler (), CSMASK_Input); to get working Axis() and Button() methods.
Implements iInputBinder.
virtual void csInputBinder::SaveConfig | ( | iConfigFile * | , | |
const char * | subsection | |||
) | [virtual] |
Save bindings to a configuration file.
Implements iInputBinder.
virtual void csInputBinder::UnbindAll | ( | ) | [virtual] |
Remove all bindings.
Implements iInputBinder.
virtual bool csInputBinder::UnbindAxis | ( | unsigned | cmd | ) | [virtual] |
Remove a binding.
Implements iInputBinder.
virtual bool csInputBinder::UnbindButton | ( | unsigned | cmd | ) | [virtual] |
Remove a binding.
Implements iInputBinder.
The documentation for this class was generated from the following file:
- csutil/binder.h
Generated for Crystal Space 2.0 by doxygen 1.6.1